Just by reading the title, I know that many people will hate me. But as this statement exists in C# I wanted to introduce it even if I honestly never used it in .NET. This is a keyword that was very useful, or even maybe essential in older languages but it then became a developer’s nightmare. C# is one which kept this legacy. This statement allows you to break the sequencential execution of a method and continue elsewhere, based on a label. Labels can be created before or after the goto statement, which means that you can go back in time. Sounds great ? And what if I tell you that this is exactly what is called spaghetti code ? That kind of code quickly becomes hard to maintain. But let’s get back to business anyway with an example. In a console, I’m asking a user to enter a number and, if the value is fine, I tell him/her whether it is an even or an odd number (Figure 1).
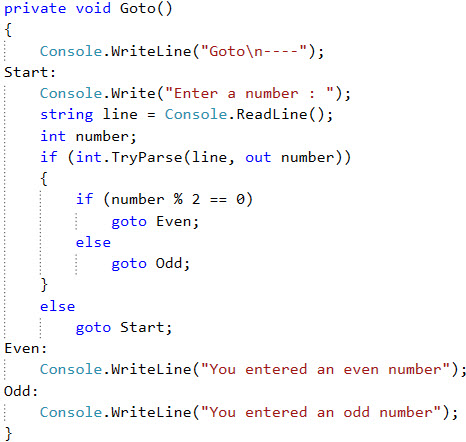
Figure 1
Let’s analyze this code. I start with a first condition to check that the entered value is correct. If it is, then I check whether the number is even or odd. In both cases I use a goto statement followed by a label name. Those labels are defined later in the method followed by a colon and statements to execute. Another label, Start, is defined at the very beginning of the method, and is called when the entered value is not correct. When a goto statement is met, the execution is directly sent to the place where the corresponding label is located. Note that I could have plcaed the statements inside the if and else blocks and the call to Start could have been replaced by a loop. But the goal here is to show you how to use the goto. Let’s see the result when I enter an odd number (Figure 2).

Figure 2
Awesome! The execution directly went to the correct statement to display the expected result. Let’s now see the call to the Start label (Figure 3).

Figure 3
Once again the result is correct. And finally just for fun, let’s see the result when I enter an even number (Figure 4).

Figure 4
Oops. What the hell happened ? Ah yeah, I forgot to tell you that labels are limited to a single line of code so all the statements that follow do not belong to it. This means that when the execution goes to a label, the program sequentially executes the statement of the label and all the ones that follow it, even if some of them are part of other labels. Thus I have to anticipate this behavior and add a label at the end of the method to which I can go when I’m in the first case (Figure 5).
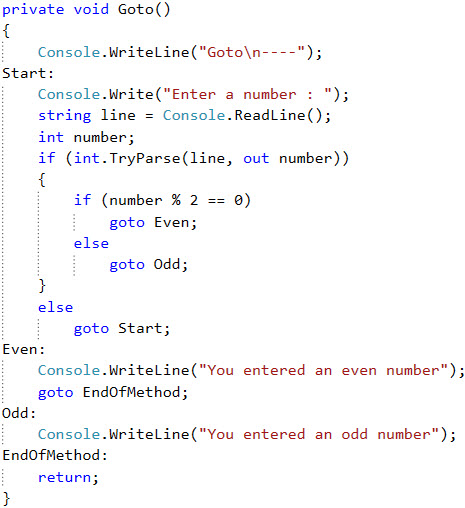
Figure 5
I added a label at the end of the method and a statement, because otherwise the code would not build. I then call this label after the Even label display is executed. If I run the application again with the number I used previously, the result is different (Figure 6).

Figure 6
Now that’s better! This time we have the good result in every case. But I hope you now understand why most developers don’t want to use the goto statement. The code quickly becomes harder to read and above all to maintain. That’s why I would advise you not to use it, unless you really like that kind of coding of course.
I will leave it at that now but I’ll write another post to show you how to use the goto in a switch. Because in that case, I think it may be useful and acceptable without creating spaghetti code.